Introduction to automated browsers using selenium
Selenium is powerful python library which mainly automates browsers which makes it immensely useful for testers to create test cases with ease and control. Unlike other testing tools or macros like grease monkey/ temper monkey which work inside browser,it has power of python at its core its strength is great when talking about resource usage, control it shows over separately opened windows/ web drivers is unmatched.
If you don’t have python set up on your computer yet then no worries I suggest you to follow this tutorial Getting started with python to catch up with no brainier tutorial of setting up python development environment in your system.
Without wasting further time, lets start with writing simple program which opens up chrome web driver with certain link and then wait for certain time to pass.
Hello world in selenium python example: working code
import time
from selenium import webdriver
from webdriver_manager.chrome import ChromeDriverManager
# URL to navigate to
url = r'https://www.google.com/search?q=cryptobool.com'
# Using WebDriverManager to automatically download and manage ChromeDriver
driver = webdriver.Chrome(ChromeDriverManager().install())
# Open the URL in the Chrome browser
driver.get(url)
# Wait for 5 seconds
time.sleep(5)
# Close the browser
driver.quit()
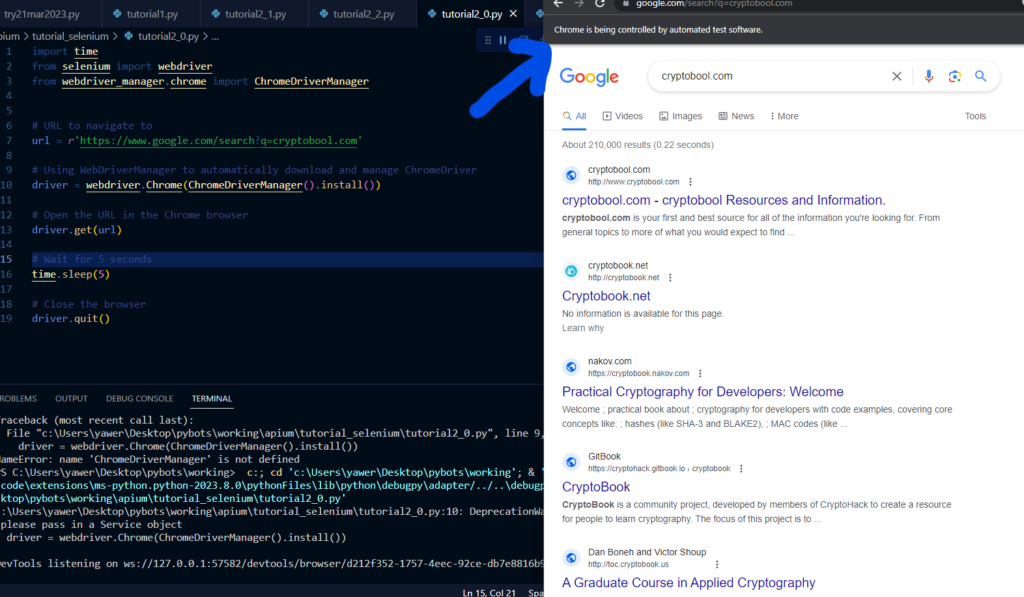
Chrome web driver would be new profile which would be created at runtime so it would treat you as new user with no previous data,so all the stored data, such as cookies, logins, history,extensions will be temporarily created in cache for the time this automated chrome browser is running and deleted once its closed.
If you want to use chrome browser with profile or data loaded already from some user of your computer then feel free to checkout this small read which will help you create automated chrome webdriver with profile of another chrome user.
Getting started In Python selenium chrome example: Explanation line by line
To break down libraries that we used so far
import time
The import time
statement allows us to use the functionalities provided by the time
module in Python’s standard library. This module provides functions to work with time-related operations, such as introducing delays, measuring time intervals, and more.
from selenium import webdriver
The from selenium import webdriver
line imports the webdriver
module from the selenium
package. Selenium is a powerful automation tool for controlling web browsers programmatically. By importing webdriver
, we gain access to the necessary classes and methods to interact with browsers and perform actions like clicking elements, filling forms, and navigating web pages.
from webdriver_manager.chrome import ChromeDriverManager
This line imports the ChromeDriverManager
class from the webdriver_manager.chrome
module. The webdriver_manager
package simplifies the management of web drivers like ChromeDriver. ChromeDriverManager
is specifically responsible for handling the installation and management of the Chrome WebDriver.
# URL to navigate to
url = r'https://www.google.com/search?q=cryptobool.com'
In this line, we define a variable url
which contains the URL of the web page we want to navigate to. In this case, it is set to https://www.google.com/search?q=cryptobool.com
, which is a Google search query for the term “cryptobool.com”.
# Using WebDriverManager to automatically download and manage ChromeDriver
driver = webdriver.Chrome(ChromeDriverManager().install())
Here, we use the webdriver
module from Selenium to create an instance of the Chrome WebDriver. By utilizing the ChromeDriverManager()
function from webdriver_manager.chrome
, we automatically download and manage the appropriate version of the ChromeDriver required to interact with the Chrome browser.
# Open the URL in the Chrome browser
driver.get(url)
This line instructs the WebDriver to open the specified URL (url
) in the Chrome browser. The get()
method is used to navigate to the provided URL.
# Wait for 5 seconds
time.sleep(5)
Here, we use the time
module’s sleep()
function to introduce a pause in the program execution. In this case, the program waits for 5 seconds before proceeding to the next line of code. It’s like taking a short break, allowing time for the web page to load or for other actions to be performed.
# Close the browser
driver.quit()
Finally, we use the quit()
method on the WebDriver instance to close the browser and end the WebDriver session. This ensures that the browser window is properly closed and any associated resources are released.
Automated download of chrome web driver: ScreenShot
Before showing this automated chrome browser, if not downloaded already it will download in terminal automatically (hardly would take couple of seconds depending upon internet connection and would look something like this).
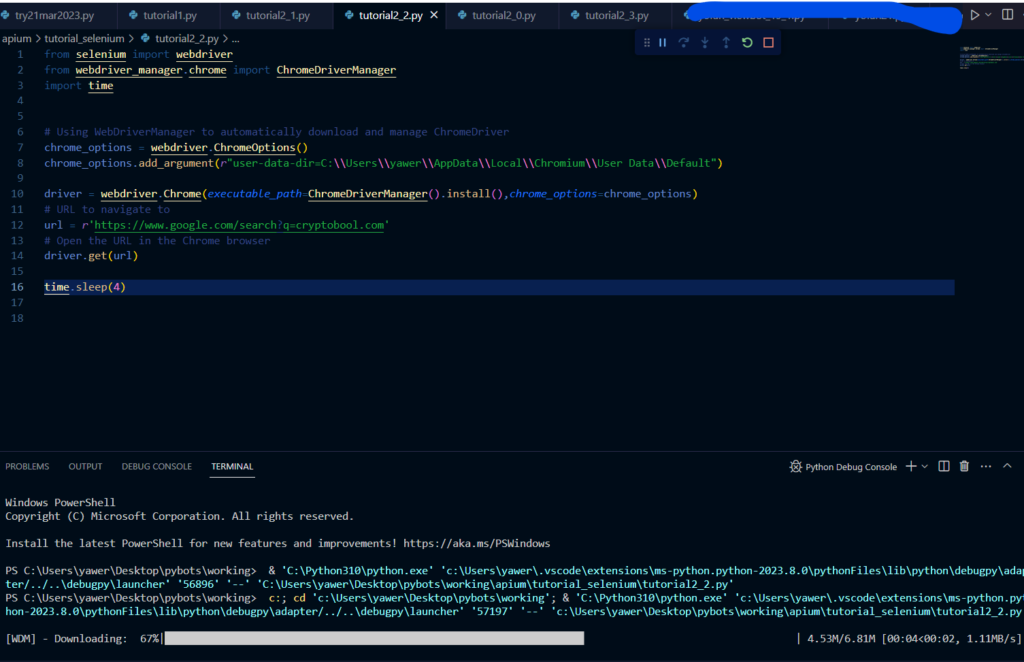
If you want to load custom profile path, with stored logins, extension from user of your computer which is also known as loading custom chrome user profile then here is the full article tutorial of loading custom profile in chrome.
I hope this precise explanation helps you understand the technical details of each line of code. Don’t be shy to ask questions in comment box and I will make sure you get answers for your hard questions. Happy coding and exploring the web!